check
Check checkbox(es) or radio(s).
caution
This element must be an <input>
with type checkbox
or radio
.
Syntax​
.check()
.check(value)
.check(values)
.check(options)
.check(value, options)
.check(values, options)
Usage​
cy.get('[type="checkbox"]').check() // Check checkbox element
cy.get('[type="radio"]').first().check() // Check first radio element
cy.check('[type="checkbox"]') // Errors, cannot be chained off 'cy'
cy.get('p:first').check() // Errors, '.get()' does not yield checkbox or radio
Arguments​
Value of checkbox or radio that should be checked.
Values of checkboxes or radios that should be checked.
Pass in an options object to change the default behavior of .check()
.
Option | Default | Description |
---|---|---|
animationDistanceThreshold | animationDistanceThreshold | The distance in pixels an element must exceed over time to be considered animating. |
log | true | Displays the command in the Command log |
force | false | Forces the action, disables waiting for actionability |
scrollBehavior | scrollBehavior | Viewport position to where an element should be scrolled before executing the command |
timeout | defaultCommandTimeout | Time to wait for .check() to resolve before timing out |
waitForAnimations | waitForAnimations | Whether to wait for elements to finish animating before executing the command. |
Yields ​
.check()
yields the same subject it was given from the previous command.
Examples​
No Args​
Check all checkboxes​
cy.get('[type="checkbox"]').check()
Select all radios​
cy.get('[type="radio"]').check()
Check the element with id of 'saveUserName'​
cy.get('#saveUserName').check()
Value​
Select the radio with the value of 'US'​
<form>
<input type="radio" id="ca-country" value="CA" />
<label for="ca-country">Canada</label>
<input type="radio" id="us-country" value="US" />
<label for="us-country">United States</label>
</form>
cy.get('[type="radio"]').check('US')
Values​
Check the checkboxes with the values 'subscribe' and 'accept'​
<form>
<input type="checkbox" id="subscribe" value="subscribe" />
<label for="subscribe">Subscribe to newsletter?</label>
<input type="checkbox" id="acceptTerms" value="accept" />
<label for="acceptTerms">Accept terms and conditions.</label>
</form>
cy.get('form input').check(['subscribe', 'accept'])
Options​
Check an invisible checkbox​
You can ignore Cypress' default behavior of checking that the element is
visible, clickable and not disabled by setting force
to true
in the options.
cy.get('.action-checkboxes')
.should('not.be.visible') // Passes
.check({ force: true })
.should('be.checked') // Passes
Find checked option​
You can get the currently checked option using the jQuery's :checked selector.
<form id="pick-fruit">
<input type="radio" name="fruit" value="orange" id="orange" />
<input type="radio" name="fruit" value="apple" id="apple" checked="checked" />
<input type="radio" name="fruit" value="banana" id="banana" />
</form>
cy.get('#pick-fruit :checked').should('be.checked').and('have.value', 'apple')
Notes​
Actionability​
The element must first reach actionability​
.check()
is an "action command" that follows all the rules of
Actionability.
Rules​
Requirements ​
.check()
requires being chained off a command that yields DOM element(s)..check()
requires the element to have typecheckbox
orradio
.
Assertions ​
.check()
will automatically wait for the element to reach an actionable state.check()
will automatically retry until all chained assertions have passed
Timeouts ​
.check()
can time out waiting for the element to reach an actionable state..check()
can time out waiting for assertions you've added to pass.
Command Log​
check the element with name of 'emailUser'
cy.get('form').find('[name="emailUser"]').check()
The commands above will display in the Command Log as:

When clicking on check
within the command log, the console outputs the
following:
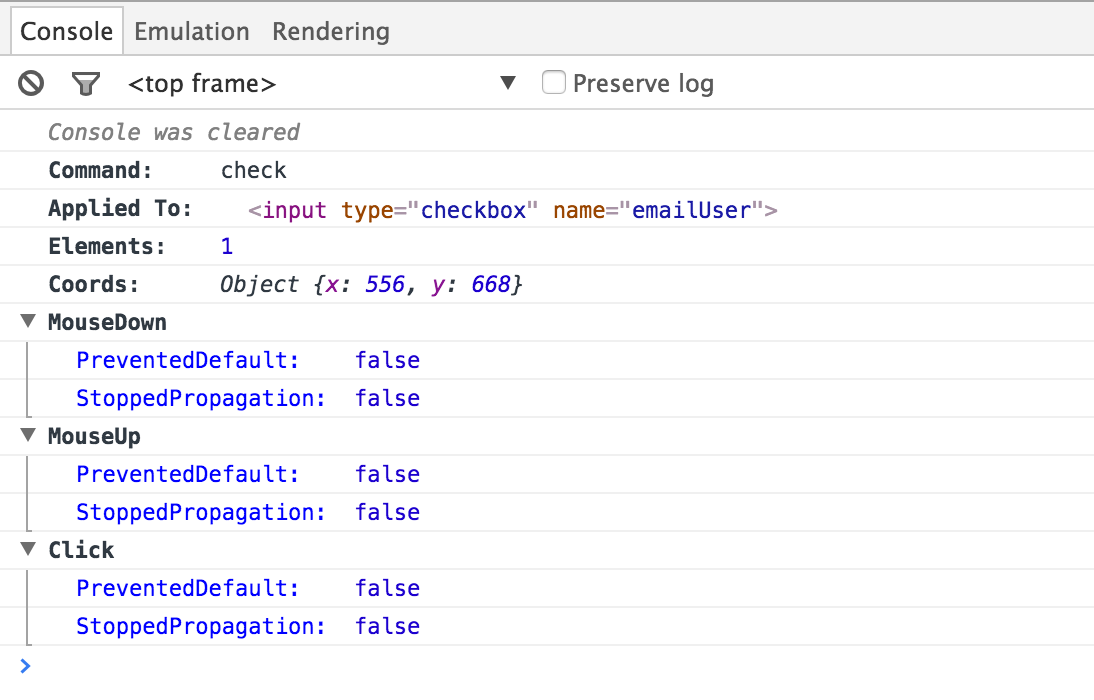